What are Push Notifications?
Push notifications are messages that are sent directly to users' mobile or desktop devices, without the need for them to open a particular application or website. These notifications appear in the form of alerts on the device screen, even if the corresponding application is not open or in use.
Push notifications are commonly used by mobile applications and web services to keep users informed about important updates, relevant events, special offers, appointment reminders, among others. Push notifications can also include a direct link to the application or website so that the user can access additional information or take immediate action.
Push notifications for customer loyalty
Push notifications are an effective tool for building customer loyalty. By sending personalized and relevant push notifications, user retention can be increased and the customer experience improved. Some ways in which businesses can use push notifications for customer loyalty include:
Offers and promotions: Push notifications can be used to send exclusive offers and promotions to loyal customers. For example, discount coupons or promotional codes can be sent to incentivize repeat purchases.
Product and service updates: Push notifications inform customers about updates and improvements to the products or services they use. These updates interest customers and help keep them engaged with the brand.
Event and appointment reminders: Push notifications remind customers about upcoming events and appointments. This can be especially useful for service-based businesses, such as beauty salons or medical clinics.
Personalized communications: Push notifications can also be used to send personalized messages, such as birthday or customer anniversary greetings. These messages can help customers feel valued and increase their loyalty to the brand.
Implementing push notifications with OneSignal in a Flutter application
OneSignal is a push notification platform that integrates easily with mobile applications and websites. Integrating OneSignal with Flutter is straightforward, and provides a simple way to send personalized push notifications to the application's users.
Let's go over the steps for implementing push notifications with OneSignal and Flutter!
OneSignal requirements
Firstly, to use OneSignal you'll need to create an account on the platform. With that out of the way, follow the prompts in the OneSignal quick start guide to create an application and obtain the necessary keys for integration with Flutter.
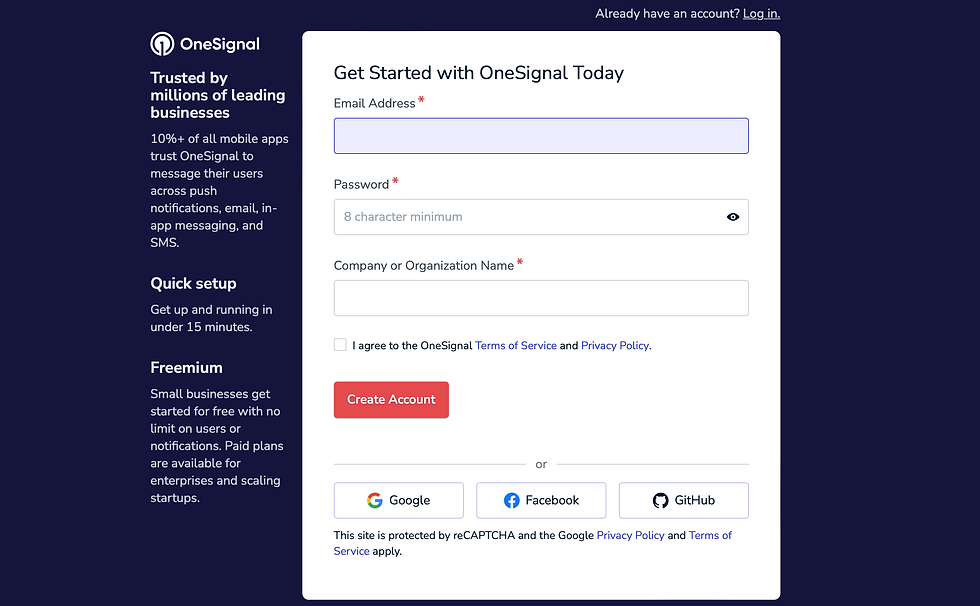
Next, you'll need to meet the following requirements to start the integration process.
Basic requirements:
OneSignal account
OneSignal ID available for use in the application, which can be found in the Keys & IDs section.
The following image shows all the sections available in the OneSignal dashboard and a sample of the platforms that can be integrated.

Requirements for integrating OneSignal on iOS
A physical test device with an operating system greater than iOS 9. The Xcode 14+ simulator with iOS 16+ is also a viable option.
Latest versions of Xcode 12 software installed.
An iOS push certificate or an Apple P8 authentication token.
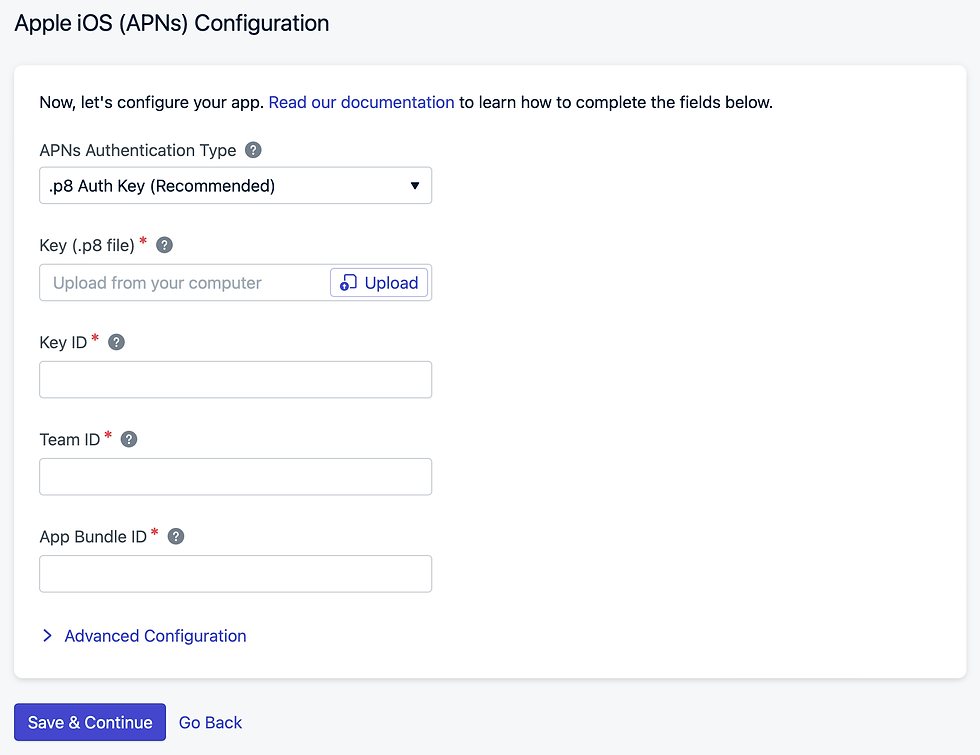
Add an iOS service extension to the corresponding section of the Flutter project. OneSignalNotificationServiceExtension allows the iOS application to receive notifications optimized with images and buttons, along with badges and confirmed deliveries. For more information on this step, we recommend consulting the official documentation provided by OneSignal.
Requirements for integrating OneSignal on Android
A device with an operating system higher than Android 4.0.3.
A user account and a Firebase project.
A Google/Firebase server API key.
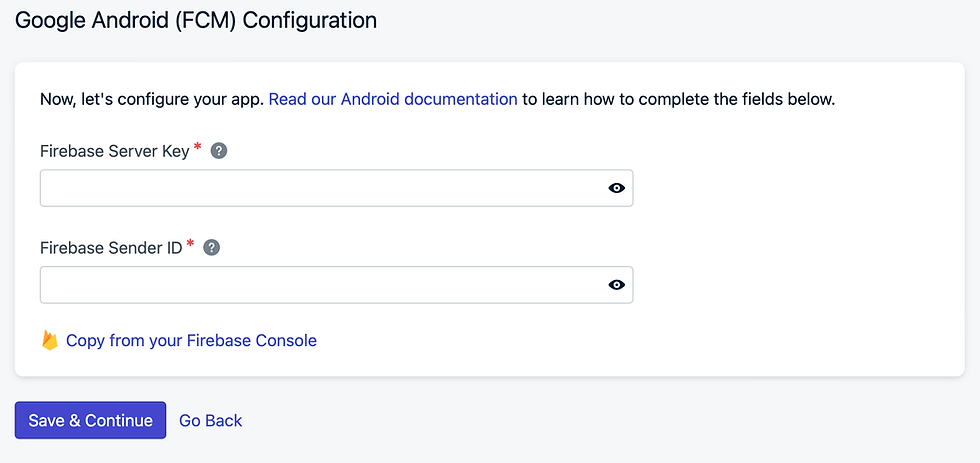
A project with a compileSdkVersion equal to version 33 or higher (higher than the default for Flutter version 2.8.0) is required. Open your android/app/build.gradle file and inspect the compileSdkVersion property.
android {
compileSdkVersion 33
...
}
OneSignal Integration in Flutter (all platforms)
To integrate OneSignal into a Flutter application, you must add the OneSignal Flutter package to the pubspec.yaml file of the application. Then, the platform needs to be initialized in the main.dart file of the application.
void main() async{
await OneSignal.shared.setAppId("YOUR_APP_ID");
...
}
Additionally, you can add viewers for various events like the receipt or opening of a new notification or to observe changes in the subscription state. Several methods that provide these functions are shown below.
The setNotificationWillShowInForegroundHandler method will be called every time a notification is received in the foreground.
OneSignal.shared.setNotificationWillShowInForegroundHandler((OSNotificationReceivedEvent event) {
event.complete(event.notification);
});
The setNotificationOpenedHandler method will be called every time a notification is opened or a button is pressed.
OneSignal.shared.setNotificationOpenedHandler((OSNotificationOpenedResult result) {
...
});
The setSubscriptionObserver method will be called every time the subscription changes, i.e., when the user registers with OneSignal and gets a user ID.
OneSignal.shared.setSubscriptionObserver((OSSubscriptionStateChanges changes) {
...
});
The setEmailSubscriptionObserver method will be called every time the user's email subscription changes, i.e., when the OneSignal.setEmail(email) method is called and the user registers.
OneSignal.shared.setEmailSubscriptionObserver((OSEmailSubscriptionStateChanges emailChanges) {
...
});
Finally, run your application on a physical device to ensure that it compiles correctly on the target platforms. Note that the iOS simulator does not support the reception of remote automatic notifications.
Check all registered users in the Audience section of the OneSignal Dashboard. Then, you can send your first notification from the OneSignal Dashboard and check its reception by registered users.